Appearance
Create template on Doppio
Whether you want to create a contract, an invoice, or a social media banner, our HTML template editor is here to help you. We also have some basic models (including some with paged.js) that will allow you to speed up the construction of your Doppio templates.
Templates will help you create documents with a consistent layout without needing to write the same HTML code over and over again.
Write your HTML once and use it as many times as you want.
Here's a short video that quickly shows you how to create your first template.
What's a Doppio template ?
A Doppio template is essentially an HTML file.
html
<!DOCTYPE html>
<html>
<body>
<h1>This a heading</h1>
<p>This a paragraph</p>
</body>
</html>
With the templates, you can create not only PDFs but also screenshots.
Below are some examples of our models.
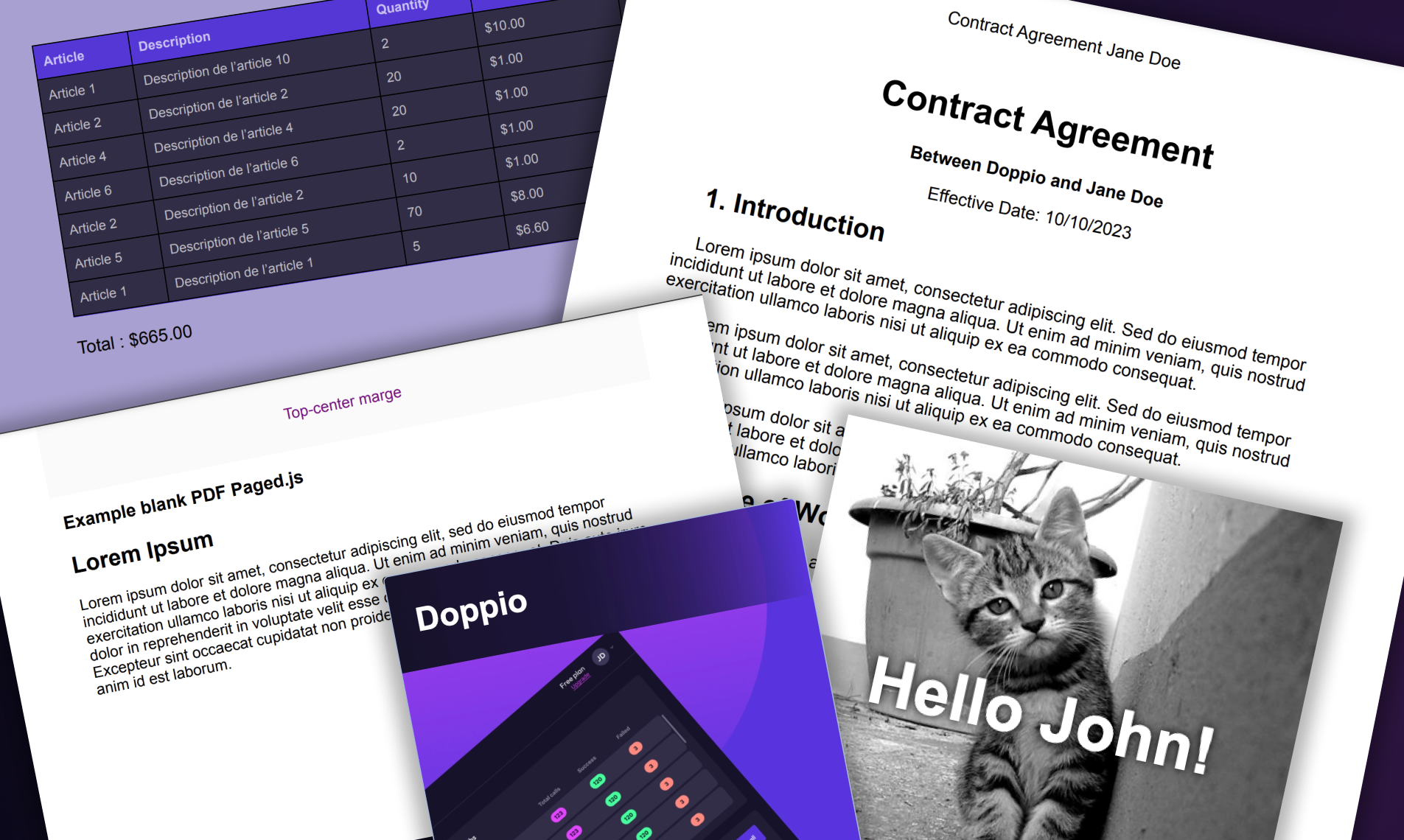
Manage templates
You can access the 'templates' menu from the logged-in area of the Doppio platform.
Here you can create, modify and delete templates in the templates section of the dashboard.
We provide you with 5 templates for creation (3 PDFs, 2 screenshots). For the PDF templates, we recommend using pagedJS, which makes it easy to manage the layout (margins, page count, ...).
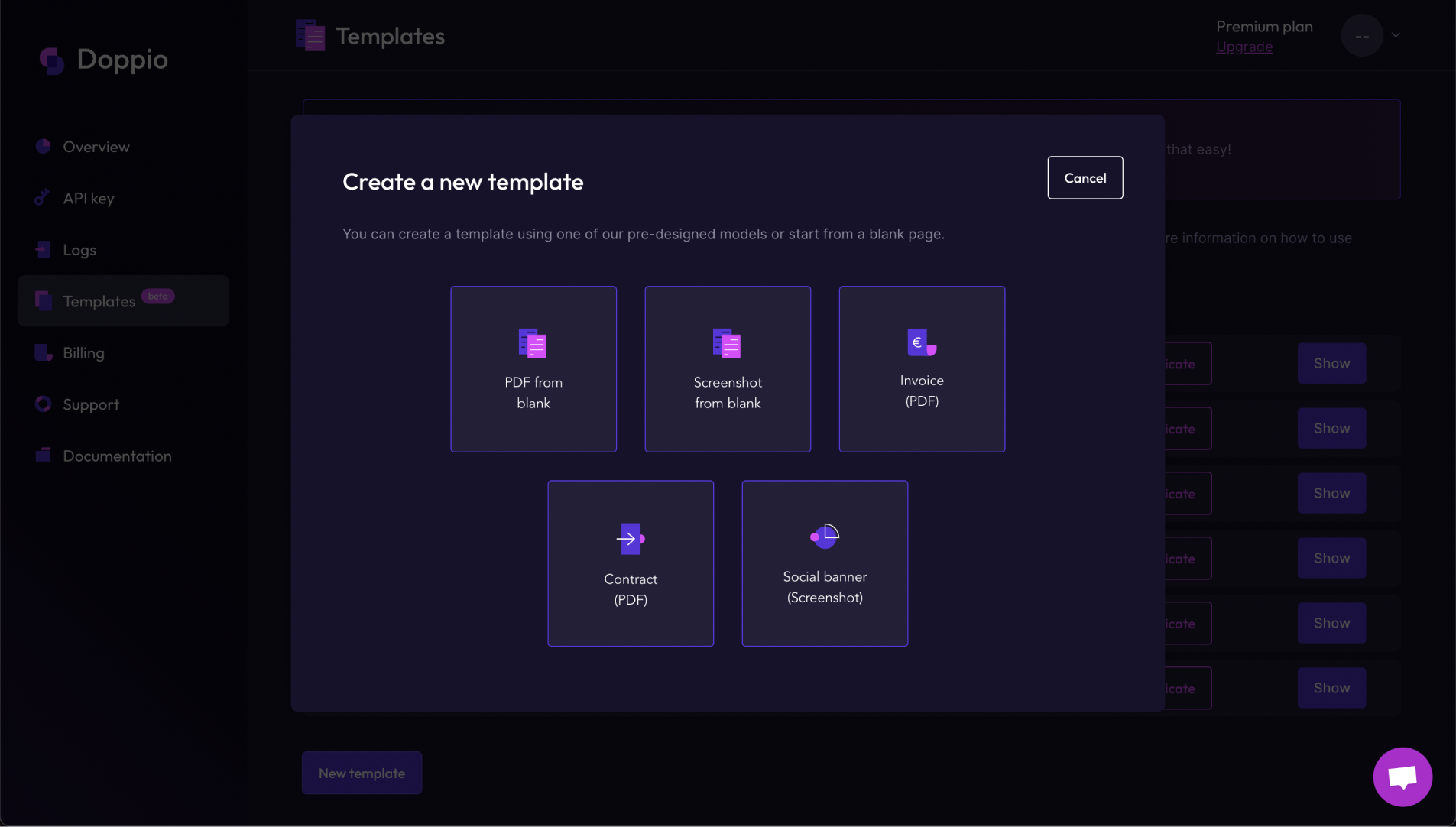
Once there, you'll access our simple HTML editor with a basic real-time preview to assist you in building your template. Additionally, by using the 'Real Doppio Render' button, you can directly view the actual Doppio rendering of the template.
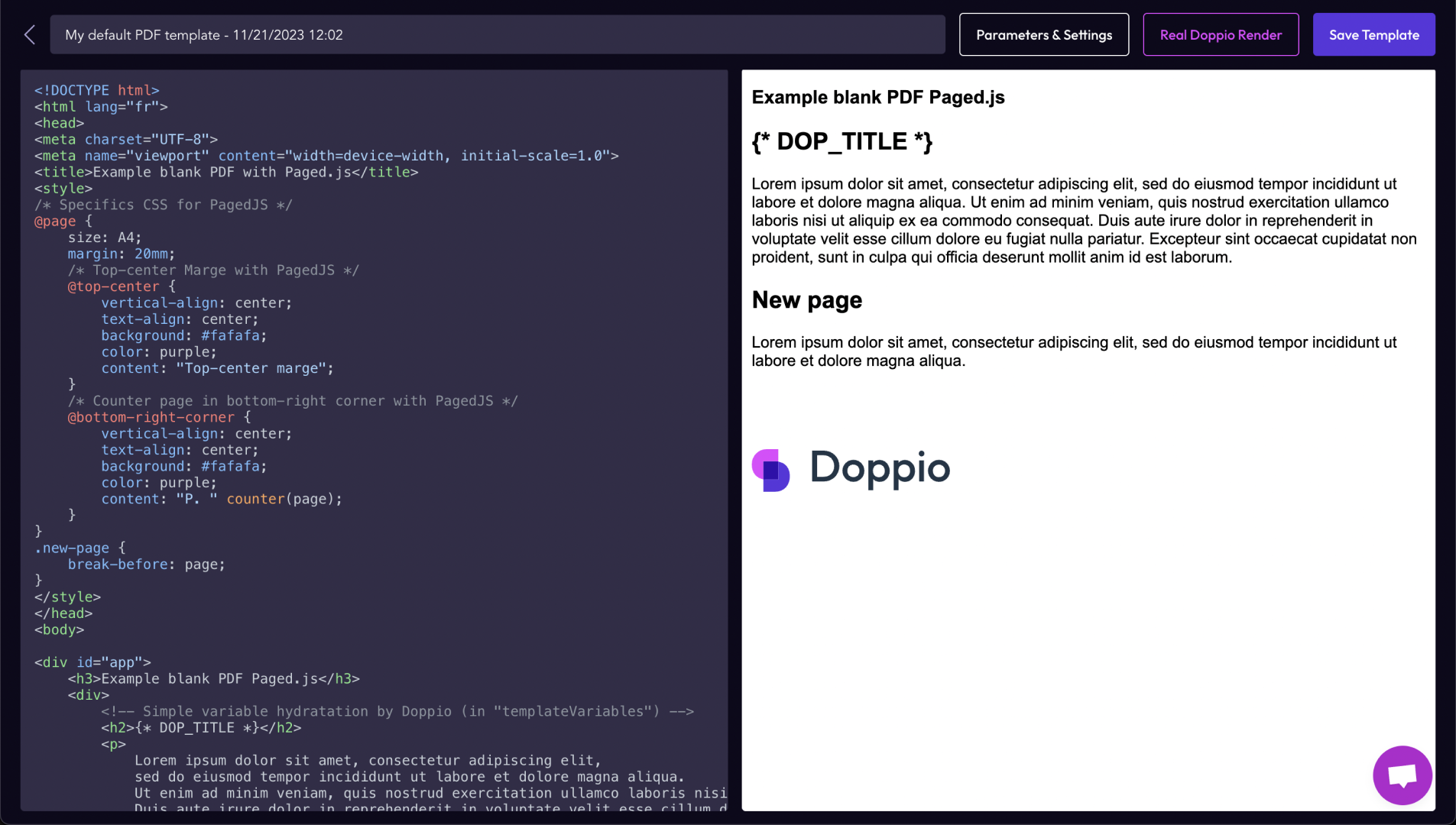
TIP
In the editor, a real-time preview is available, allowing you to see the changes made instantly. However, please note that JavaScript is not executed in this preview.
The real Doppio rendering can be viewed directly in the browser in a new tab.
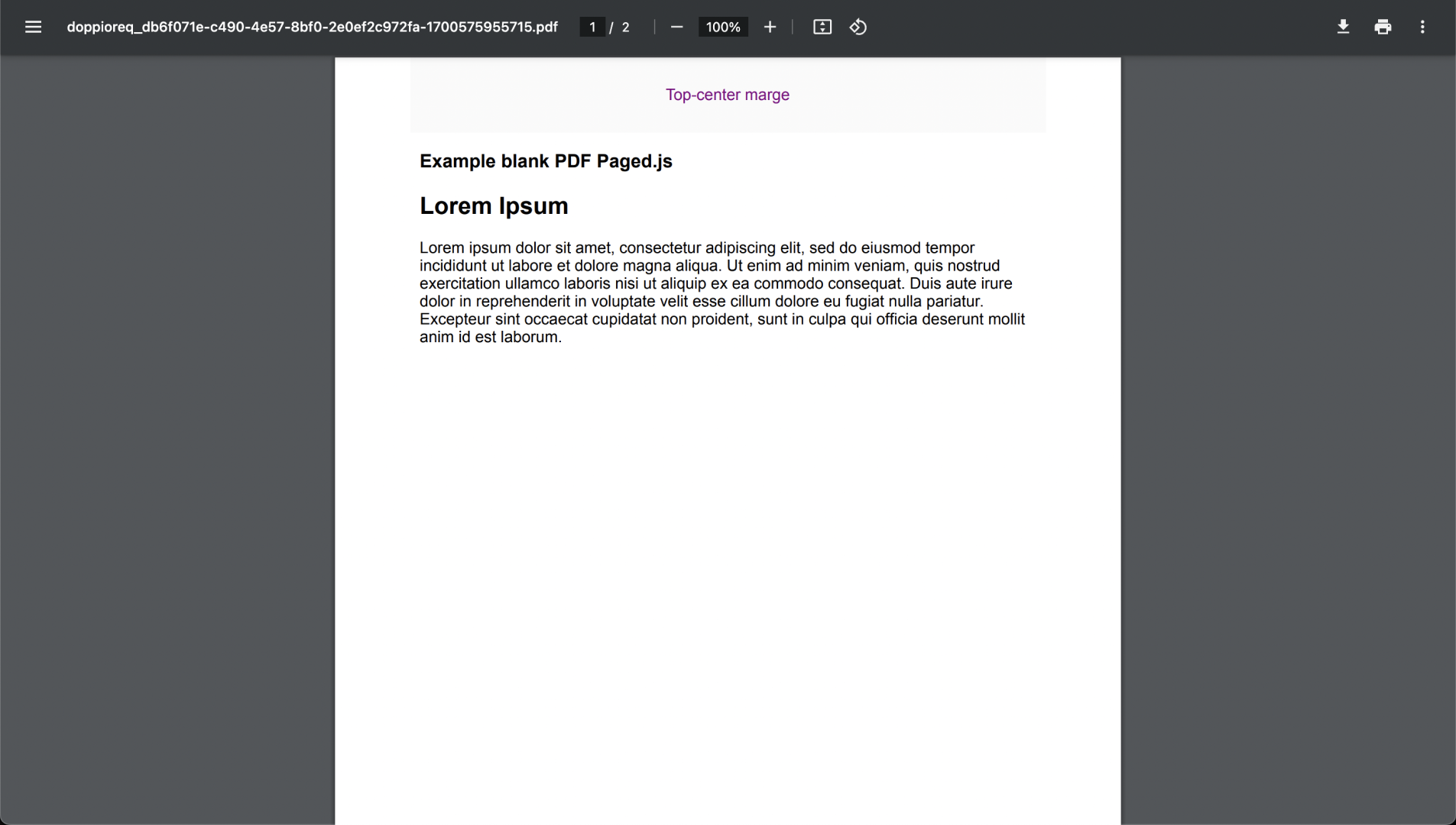
Custom variables
Templates can contain custom variables that will be replaced at render time. You can use the following syntax to create a variable in your template. All variables should start with "DOP_".
{* DOP_VARIABLE *}
As an example, let's add two variables headingName
and paragraphName
in the template :
html
<!DOCTYPE html>
<html>
<body>
<h1>This a {* DOP_HEADING *}</h1>
<p>This a {* DOP_PARAGRAPH *}</p>
</body>
</html>
You can manage default values for these variables directly in the 'Parameters & Settings' section.
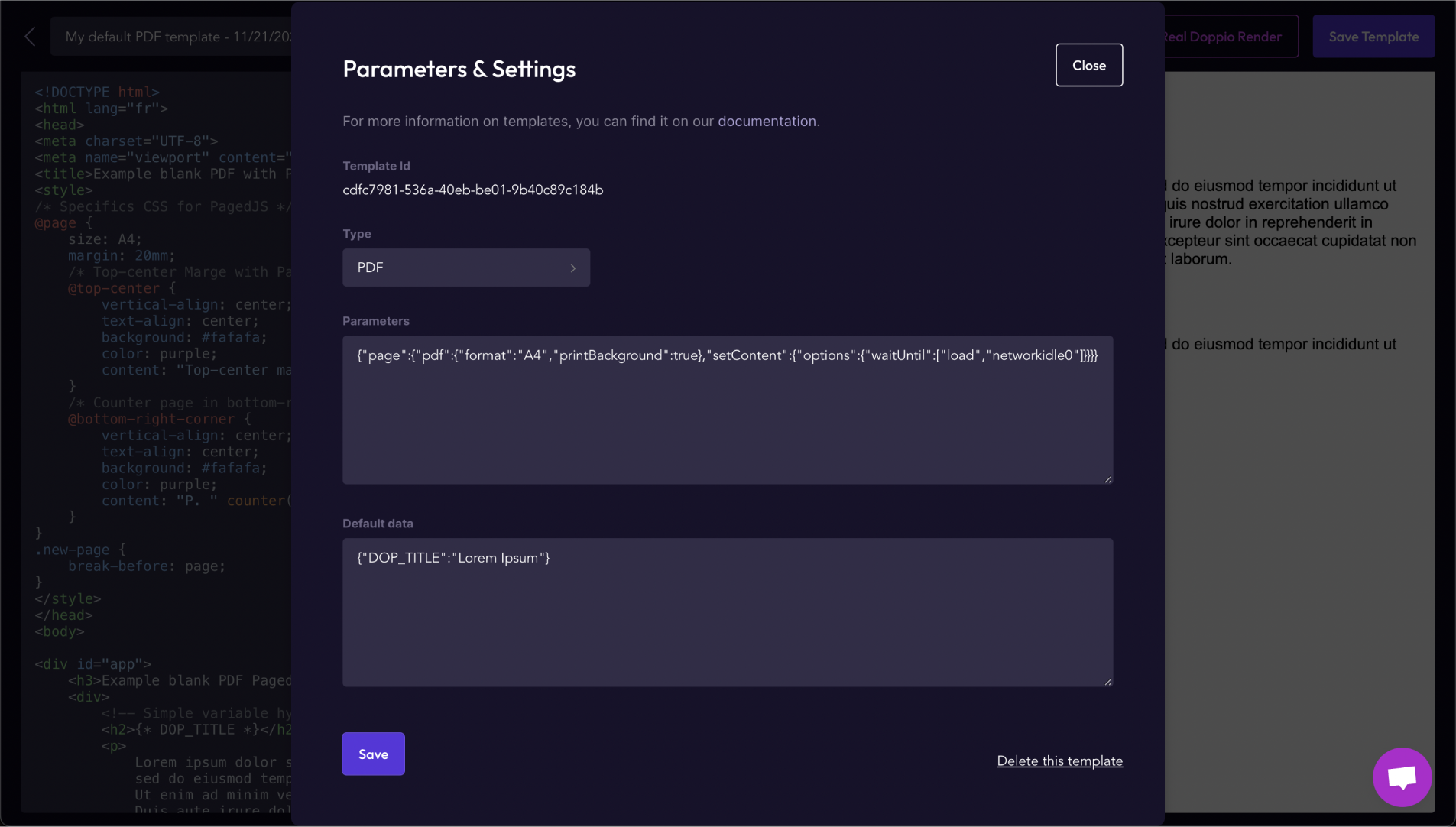
Type & Parameters
Templates also give you the possibility to add custom parameters to your document so you don't have to specify them at render time.
Here are some possible parameters for PDF:
json
{
"page": {
"pdf":{
"printBackground": true,
"format": "A4",
"width": "100px",
"height": "100px",
"margin": {
"top":"50px",
"left":"50px",
"right":"50px",
"bottom":"50px"
}
},
"setContent": {
"options": {
"waitUntil": ["networkidle2"]
}
}
}
}
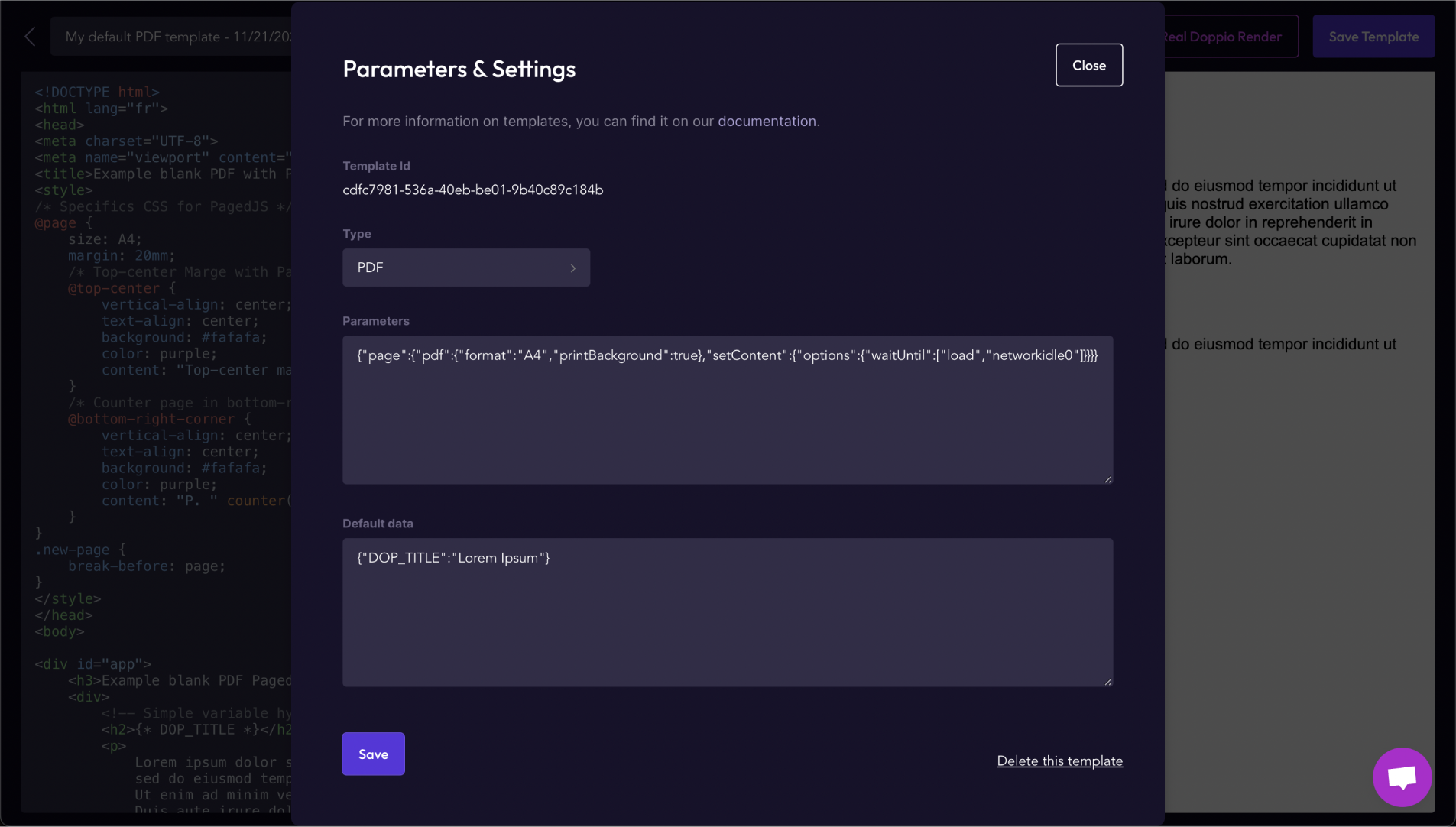
You can save with your template the parameters expected for any rendering method, see here for more informations.
How to use templates ?
You can use templates with any render method: direct, sync and async (you can find complete information about API calls for templates here).
Specify which template you want to use with the templateId
property.
And specify the values of the custom variables with the templateData
property.
You still can specify parameters at render time, they will override the ones saved with the template.
js
const options = {
method: 'POST',
url: 'https://api.doppio.sh/v1/template/direct',
headers: {
'Authorization': 'Bearer <YOUR API KEY>',
'Content-Type': 'application/json'
},
body: JSON.stringify({
templateId: '<YOUR TEMPLATE ID>',
templateData: {
"DOP_HEADING": "Heading Title",
"DOP_PARAGRAPH": "Paragraph Text"
}
})
};
const request = require('request');
request(options, function (error, response) {
if (error) throw new Error(error);
});
The rendering method will then use your template to render the document and replace your custom variables with the values you specified.
🙌 And there you have it, you can now use your first Doppio template in your code.
Feel free to provide feedback if you have any comments about the templates. Remember, we are still in beta and highly value your input!